生产者
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
| //生产者写入内存
#include <iostream>
#include <unistd.h>
#include <sys/types.h>
#include <sys/shm.h>
#include <cstring>
struct bufferPool
{
char buffer[5][100];
int Index[5]; // 5个养猪槽
};
using namespace std;
int main(){
int running = 1;
void * p = NULL; // p准备指向养猪槽
struct bufferPool * shared;
char buffer[100];
int shmid; //共享猪圈ID
shmid = shmget((key_t)1234,sizeof(struct bufferPool),0666 | IPC_CREAT);
if (shmid == -1)
{
fprintf(stderr,"Error occured when getting shared ID");
exit(-1);
}
p = shmat(shmid,(void*)0,0);
if (p == (void*)-1)
{
fprintf(stderr,"Error occured when getting shared ID");
exit(-1);
}
shared = (struct bufferPool*)p;
while (running)
{
int index = 0;
INC_LABEL:
if (shared->Index[index] ==1)
{
printf("Shared Buffer %d is in use. Go to the next.\n",index);
sleep(1);
index++;
if (index == 5)
{
index = 0;
}
goto INC_LABEL;
}
else
{
//开始投食
printf("你要投什么到 %d 中:",index);
fgets(buffer,100,stdin);
strncpy(shared->buffer[index],buffer,100);
shared->Index[index]=1;
if (strncmp(buffer,"end",3)==0)
{
running = 0;
}
}
}
if (shmdt(p) == -1)
{
fprintf(stderr,"猪圈打扫失败");
exit(-1);
}
if (shmctl(shmid,IPC_RMID,0) == -1){
fprintf(stderr,"猪圈没关门");
exit(-1);
}
exit(0);
return 0;
}
|
消费者
//生产者写入内存
#include <iostream>
#include <unistd.h>
#include <sys/types.h>
#include <sys/shm.h>
#include <cstring>
struct bufferPool
{
char buffer[5][100];
int Index[5]; // 5个养猪槽
};
using namespace std;
int main(){
int running = 1;
void * p = NULL; // p准备指向养猪槽
struct bufferPool * shared;
char buffer[100];
int shmid; //共享猪圈ID
shmid = shmget((key_t)1234,sizeof(struct bufferPool),0666 | IPC_CREAT);
if (shmid == -1)
{
fprintf(stderr,"Error occured when getting shared ID");
exit(-1);
}
p = shmat(shmid,(void*)0,0);
if (p == (void*)-1)
{
fprintf(stderr,"Error occured when getting shared ID");
exit(-1);
}
shared = (struct bufferPool*)p;
while (running)
{
int index = 0;
INC_LABEL:
if (shared->Index[index] != 1)
{
printf("%d 没食物了,去下一个看看.\n",index);
sleep(rand()%3);
index++;
if (index == 5)
{
index = 0;
}
goto INC_LABEL;
}
else
{
//开始吃东西
printf("猪猪开始吃 %d 食物\n",index);
shared->Index[index]=0;
printf("我吃到了:%s",shared->buffer[index]);
if (strncmp(buffer,"end",3)==0)
{
running = 0;
}
}
}
if (shmdt(p) == -1)
{
fprintf(stderr,"猪圈打扫失败");
exit(-1);
}
if (shmctl(shmid,IPC_RMID,0) == -1){
fprintf(stderr,"猪圈没关门");
exit(-1);
}
exit(0);
return 0;
}
效果图
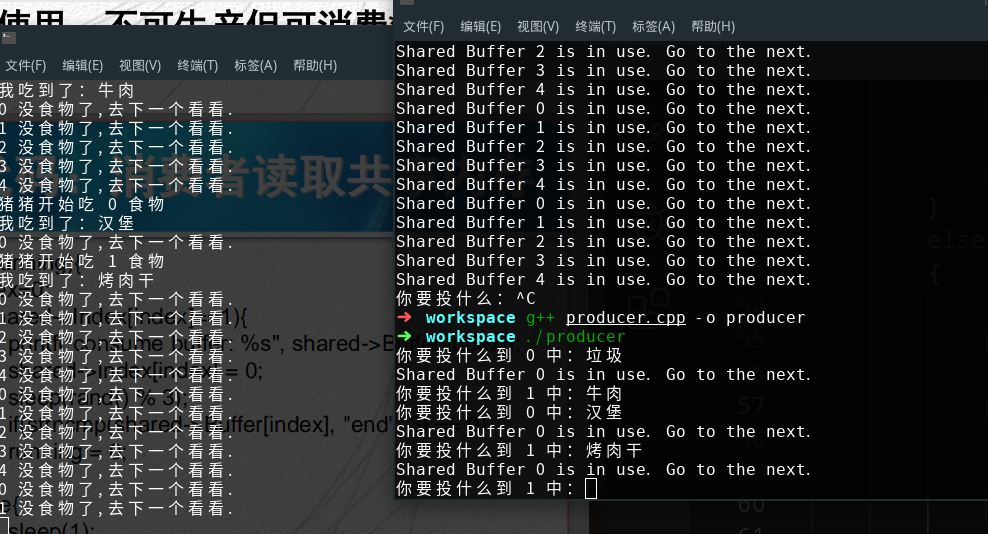